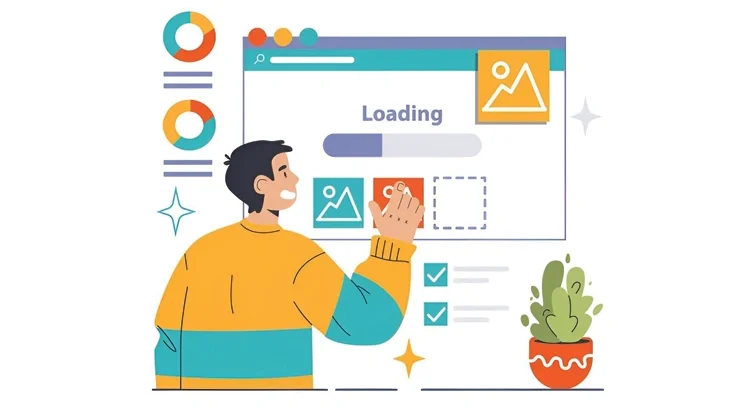
Optimizing JavaScript Performance: Tips and Techniques
In today’s web landscape, JavaScript reigns supreme as the language that injects dynamism and interactivity into static web pages. While it empowers captivating user experiences, optimized JavaScript can bring them crashing down–resulting in sluggish performance and frustrated users. This comprehensive guide dives deep into effective strategies and techniques to optimize your JavaScript for a seamless and performant user experience.
The Performance Imperative
Prioritizing performance is no longer optional–it’s a fundamental tenet of modern web development. JavaScript execution can impact a web page’s load time and responsiveness. Studies have shown a direct correlation between slow loading times and user abandonment. By optimizing your JavaScript, you make sure your web application delivers a smooth and engaging experience, keeping users satisfied and coming back for more.
Core Strategies for Optimization
Optimizing JavaScript performance is a multi-faceted approach that requires considering various factors. Here, we’ll explore the key areas to focus on:
01. Minimizing DOM Manipulation:
The Document Object Model (DOM) is the structure of your web page. Frequent DOM manipulation, like adding, removing, or changing elements, can be expensive. This can lead to rendering bottlenecks and sluggish performance. Here’s how to tackle this:
Reduce DOM Access: Instead of querying the DOM for elements, store references to use elements in variables. This minimizes the number of times the browser needs to traverse the DOM tree, improving efficiency.
Batch Updates: If you need to make multiple DOM modifications, group them and do them in a single batch. This reduces re-flows and repaints, which are expensive operations that the browser performs to update the layout and rendering of the page.
Consider Virtual DOM Libraries: For complex applications with frequent DOM updates, consider using virtual DOM libraries like React or Vue.js. These libraries create a lightweight, in-memory representation of the DOM, allowing for efficient updates before synchronizing them with the current DOM.
02. Leveraging Browser Caching:
Modern browsers offer robust caching mechanisms that can improve performance, especially for returning visitors. By caching static JavaScript files (those that don’t change), the browser can avoid downloading them on later visits, leading to faster page loads.
HTTP Caching Headers: Leverage HTTP caching headers like Cache-Control and Expires to instruct the browser on how long to cache resources. This allows the browser to reuse cached resources instead of downloading them again.
Browser Caching Libraries: Several JavaScript libraries simplify browser caching management. These libraries offer an API for setting up caching headers and managing cache invalidation strategies.
03. Minification and Compression:
Minification involves removing unnecessary characters like whitespace, comments, and formatting from your JavaScript code. This results in smaller file sizes, which translates to faster download times. Compression techniques like Gzip further reduce the file size by encoding the code, leading to even faster downloads. Most build tools and deployment pipelines can minify and compress your JavaScript code.
Optimizing Code Structure
Beyond these foundational practices, consider these code-level optimizations for a performance boost:
Choosing the Right Data Structures:
The choice of data structures like arrays or objects can significantly impact performance. For example, if you need frequent random access, arrays might be preferable. If you primarily need to perform lookups by key, objects might be more suitable. Select the most appropriate data structure for your use case, considering factors like access patterns and mutability.
Writing Efficient Algorithms:
Algorithmic complexity plays a major role in JavaScript performance. Strive for algorithms with lower time and space complexity. For instance, a linear search algorithm (iterating through each element in an array) is less efficient than a binary search algorithm (dividing the search space in half with each iteration) for sorted arrays. Consider utilizing built-in JavaScript methods and libraries optimized for common operations like sorting, searching, and filtering.
Avoiding Global Variables:
Global variables can lead to unintended side effects and make code harder to maintain. By using local variables within functions or modules, you improve code clarity and avoid potential performance issues caused by unintended global variable modifications.
Advanced Techniques for Complex Applications
As your web application matures and scales, you might explore more advanced techniques for further optimization:
01. Code Splitting:
Code splitting involves breaking down your JavaScript code into smaller bundles. These bundles can be loaded on-demand only when the user navigates to a specific section of the application or interacts with a particular feature. This reduces initial load times and improves perceived performance, especially for larger applications. Modern bundlers like Webpack can automate code splitting based on route configurations or code dependencies.
02. Lazy Loading:
As mentioned earlier, lazy loading defers the loading of non-critical resources like images or JavaScript files until they are required by the user. This prioritizes the loading of essential content for the initial page view, leading to a faster perceived load time. Here’s how it works:
Image Lazy Loading: Techniques like Intersection Observer API or custom scroll event listeners can be used to identify when an image enters the viewport. The image is then dynamically loaded and displayed, ensuring users see the most crucial content first.
JavaScript Lazy Loading: Similar to images, JavaScript modules or chunks can be loaded on-demand. Libraries like RequireJS or import statements with dynamic loading can be used to achieve this. This ensures only the JavaScript needed for the current user interaction is downloaded and executed.
03. Web Workers:
Web Workers are a powerful feature that allows you to run JavaScript in background threads, freeing up the main thread for critical tasks like rendering the UI and handling user interactions. This is particularly beneficial for long-running or computationally intensive operations like complex data processing, image manipulation, or background calculations. Here are some key considerations for Web workers:
Communication: Web workers communicate with the main thread through message passing. This requires careful design to avoid race conditions and ensure data consistency.
Use Cases: Web Workers are ideal for tasks that don’t rely on the DOM or manipulate the UI directly. Examples include performing large calculations, fetching data from APIs, or running simulations.
04. WebAssembly (WASM):
Web Assembly is a low-level assembly language designed to run within web browsers. While it has a steeper learning curve compared to JavaScript, WASM offers significant performance benefits for computationally intensive tasks. Here’s why WASM might be useful:
Near-Native Performance: WASM code can be compiled from various languages like C++ or Rust, allowing for near-native performance compared to interpreted JavaScript. This is ideal for complex calculations, graphics processing, or running legacy code in the browser.
Integration with JavaScript: WASM can interact with JavaScript, allowing you to leverage the strengths of both languages. JavaScript can handle UI interactions and data management, while WASM takes over computationally heavy tasks.
05. Server-Side Rendering (SSR):
While traditionally associated with SEO benefits, Server-Side Rendering (SSR) can also improve perceived performance for complex applications. With SSR, the initial HTML content is pre-rendered on the server, including any necessary JavaScript to render the initial view. This allows for a faster initial page load and a more interactive experience before client-side JavaScript takes over.
06. Offline Capabilities:
For applications that require functionality even without an internet connection, consider implementing offline capabilities. Techniques like Service Workers and IndexedDB enable the caching of essential data and resources, allowing users to interact with a limited set of features even when offline. This can significantly improve user experience in situations with unreliable internet connectivity.
Choosing the Right Techniques
The choice of advanced techniques depends on the specific needs of your application. Here are some general guidelines:
Start with Core Optimizations: Before diving into advanced techniques, ensure you’ve implemented core optimization strategies like minimizing DOM manipulation, leveraging caching, and writing efficient code. These foundational practices offer significant performance gains in most cases.
Measure and Analyze: Continuously measure your application’s performance using browser developer tools and performance profiling techniques. Identify bottlenecks and prioritize optimizations that address the areas with the most significant impact.
Complexity vs. Benefit: Weigh the complexity of implementing an advanced technique against the expected performance gains. Don’t introduce unnecessary overhead if simpler solutions can achieve the desired performance improvement.
Remember, optimization is an ongoing process. By continuously monitoring performance, understanding user behavior, and strategically applying these techniques, you can ensure your complex JavaScript application delivers a smooth and exceptional user experience.