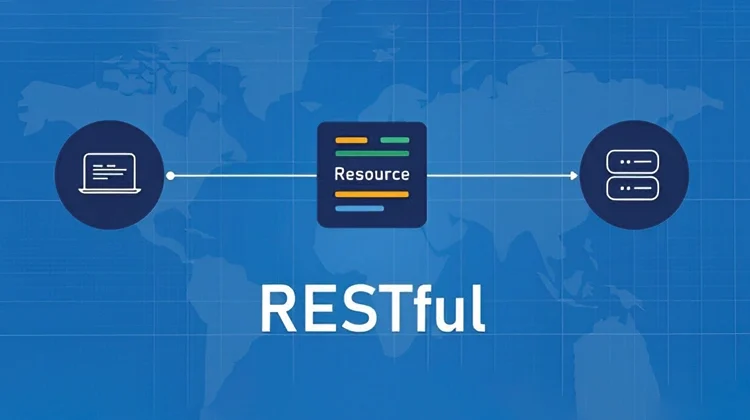
Creating RESTful APIs for Web Applications: The Comprehensive Guide
In the dynamic landscape of web development, seamless communication and data exchange between applications are no longer a luxury but a necessity. RESTful APIs (Application Programming Interfaces) have emerged as the go-to solution, providing a standardized and versatile approach to establishing communication channels. By creating RESTful APIs for web applications, developers unlock a treasure trove of benefits, including:
- Enhanced Scalability: APIs enable modular application design, allowing independent scaling of the front-end and back-end components. This fosters flexibility and simplifies handling increased traffic or data volume.
- Simplified Maintenance: Well-designed APIs encapsulate core functionalities, making maintenance and updates easier. Changes to the back-end logic can be implemented without affecting the front-end application, streamlining the development process.
- Rich User Experiences: APIs empower developers to leverage functionalities from external applications, enabling the creation of feature-rich and dynamic user experiences within their web applications.
- Expanded Market Reach: APIs can expose functionalities to a wider developer audience, fostering innovation and leading to the creation of new applications or integrations that further enhance the value proposition of your web application.
This comprehensive guide delves into the world of crafting RESTful APIs for web applications. We’ll explore the foundational concepts, delve into best practices, and give actionable tips to make sure your API is robust, secure, and developer-friendly.
Demystifying REST: The Architectural Cornerstone
REST, an acronym for Representational State Transfer, is an architectural style that dictates how APIs are designed. It emphasizes a resource-based approach, where data is accessed and manipulated through well-defined resources. These resources are identified by URIs (Uniform Resource Identifiers) and interacted with using standard HTTP methods like GET, POST, PUT, and DELETE. This standardized approach fosters clear communication between applications and simplifies the integration process.
Here’s a breakdown of the core REST principles:
- Client-Server Model: The API functions as a server, responding to requests started by client applications. This separation of concerns promotes loose coupling and facilitates independent development and deployment of applications.
- Stateless Communication: Each ask from a client to the server must contain all the information for processing. The server doesn’t keep any session state between requests, ensuring scalability and simplifying server-side logic.
- Resource-Based: Data is accessed and manipulated through well-defined resources. These resources represent entities within your application domain, such as users, products, or orders.
- Uniform meet: Standard HTTP verbs are used to interact with resources. GET retrieves data, POST creates new resources, PUT updates existing resources, and DELETE removes resources.
By adhering to these principles, RESTful APIs offer a clear and consistent way for developers to interact with your web application’s data and functionalities.
Planning Your API: A Blueprint for Success
Before diving headfirst into code, meticulous planning is essential for creating a well-designed and user-friendly API. Here’s a roadmap to guide you through this crucial stage:
1. find Resources: The cornerstone of your API lies in the resources it exposes. Consider the core entities your application manages. This could encompass user data on a social media platform, product information in an e-commerce store, or blog posts in a content management system.
2. Design URIs: Craft intuitive URIs that reflect the resources they represent. These URIs should be human-readable and follow a consistent structure. For instance, /users could retrieve a list of users, while /users/123 could target a specific user with ID 123. Avoid cluttering URIs with verbs or parameters that should reside within the request body.
3. Define Data Formats: Select a format for representing data exchanged through the API. JSON (JavaScript Object Notation) is a popular choice due to its human-readable nature, ease of integration with various programming languages, and widespread adoption within the developer community. XML (Extensible Markup Language) is another option, but its verbosity can make it less suitable for modern API design.
4. Plan for CRUD Operations: RESTful APIs typically adhere to CRUD principles (Create, Read, Update, Delete). Define how each HTTP method will map to these operations on your resources. For example, a GET request to /users might retrieve a list of users, while a POST request to /users with user data in the request body might create a new user.
5. Versioning: APIs are likely to evolve. Implement a versioning strategy to allow developers to continue using older versions of your API while you introduce new features or functionalities. Common approaches include path versioning (e.g., /users/v1/all) and query parameter versioning (e.g., /users?version=1).
6. Error Handling: Robust error handling is vital for a smooth developer experience. Implement a consistent error response format that includes an HTTP status code, a clear error message, and any relevant debugging information. Standardize HTTP status codes to convey the nature of the error (e.g., 400 Bad Request, 404 Not Found, 500 Internal Server Error).
7. Pagination: When dealing with large datasets, returning all results in a single response can be overwhelming for client applications and inefficient in terms of network usage. Implement pagination mechanisms to allow developers to retrieve data in smaller chunks. This can be achieved using query parameters to specify the page number and number of items per page.
8. Filtering and Sorting: Empower developers to refine their requests by including filtering and sorting capabilities. Allow filtering based on specific resource attributes using query parameters. Sorting can be facilitated by incorporating sorting parameters within the request URI.
9. Rate Limiting: To safeguard your server from excessive traffic and potential abuse, consider implementing rate-limiting mechanisms. This can involve limiting the number of requests a client can make within a specific time frame.
10. Documentation is key: Comprehensive API documentation is paramount for successful adoption by developers. Invest time in creating clear and concise documentation that covers all aspects of your API. This includes:
- API endpoints with detailed descriptions of their functionalities
- Request and response formats, including data models and examples
- Authentication and authorization mechanisms
- Error handling codes and their meanings
- Use examples and code samples in various programming languages
Additional Considerations:
- Security: Since APIs act as gateways to your application’s data, prioritize robust security measures. Implement authentication mechanisms to verify user identities and authorization rules to control access to specific resources. Industry-standard practices like token-based authentication (JWT) are excellent choices to consider.
- Testing: Rigorous testing is crucial to ensure your API functions as expected. Utilize testing tools to simulate various scenarios, including edge cases and error conditions. Consider unit testing individual API endpoints and integration testing to verify how your API interacts with the broader application ecosystem.
By meticulously planning your API with these considerations in mind, you’ll lay a solid foundation for a well-designed and developer-friendly interface. Remember, a well-crafted API not only empowers your web application but also fosters a thriving developer community around it.