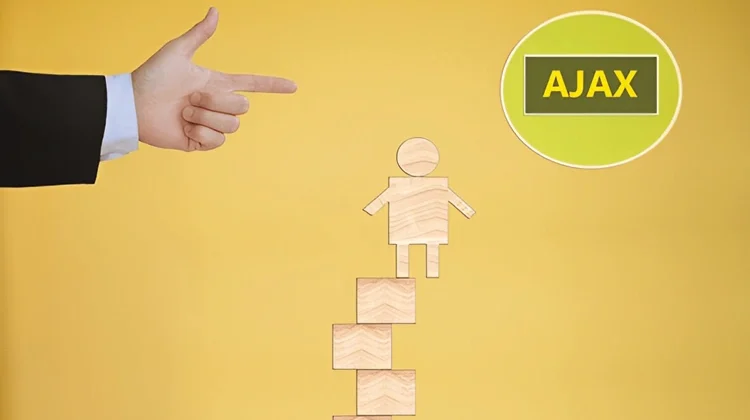
Conquering Asynchronous JavaScript: A Guide to Promises and Async/Await
JavaScript’s versatility extends to handling both synchronous and asynchronous operations. Synchronous operations are executed in the order they’re written, one after another. Asynchronous operations, but don’t block the main thread while they’re running. This is crucial in web development, where user interaction or data retrieval from servers shouldn’t freeze the entire page.
Asynchronous JavaScript can be a double-edged sword. While it enables non-blocking functionality, managing it requires the right tools. In the past, callbacks were the primary method, but their nested structure, often called “callback hell,” could make code difficult to understand and keep.
Promises introduced in ES6 (ECMAScript 2015) emerged as a game-changer for asynchronous programming in JavaScript. They give a cleaner approach to dealing with the outcome of asynchronous operations–either successful resolutions or errors.
Understanding Promises
Promises are object-based representations of the eventual completion (or failure) of an asynchronous operation. They offer a structured way to handle the result of such operations, improving readability and maintainability compared to callbacks.
Creating Promises:
Promises are created using the Promise constructor. This constructor takes an executor function with two arguments: resolve and reject. These functions are used to show whether the asynchronous operation has been successful or failed.
JavaScript
function fetch data (URL) { return new Promise (resolve, reject) => { const xhr = new XMLHttpRequest (); xhr.open(‘GET’, url); xhr. onload = () => { if (xhr. status === 200) { resolve (JSON. parse (xhr. responseText)); } else { reject (new Error (`didn’t fetch data: ${xhr. statusText}`)); } }; xhr. onerror = reject; xhr. send (); }); }
In this example, the fetchData function takes a URL and returns a promise. The executor function makes an XHR ask for the URL. If successful, it resolves the promise with the parsed JSON data using the resolve function. If there’s an error, it rejects the Promise with an error object using the reject function.
Consuming Promises:
Promises give methods for attaching callback functions that will be executed based on the outcome (resolved or rejected) of the asynchronous operation. The most commonly used methods are:
•. then (): This method takes a callback function that executes when the promise is resolved. The callback function can receive the resolved value (data) as an argument.
JavaScript
fetchData (‘https://api.example.com/data’). then (data => { console.log (data); });
•. catch (): This method takes a callback function that executes when the promise is rejected. The callback function can receive a rejecting reason (error) as an argument.
JavaScript
fetchData (‘https://api.example.com/data’). then (data => { console.log (data); }). catch (error => { console. error (error); });
Chaining Promises:
Promises allow for chaining, a powerful technique for handling sequences of asynchronous operations. Chaining involves using. Then () is the method to return another promise from the callback function. This enables executing the next asynchronous operation only after resolving the earlier one.
JavaScript
fetchData (‘https://api.example.com/data’). then (data => { return anotherAsyncFunction (data); }). then (processedData => { console.log (processedData); }) .catch(error => { console.error(error); });
In this example, fetchData retrieves data, then anotherAsyncFunction processes it. The company finally logs the processed data, while the catch handles any errors.
Error Handling with Promises:
Error handling is a crucial aspect of asynchronous programming. Promises provide a structured approach to managing errors using the .catch() method. This method allows you to define a callback function that will be executed if any of the Promises in the chain are rejected.
JavaScript
fetchData(‘https://api.example.com/data’) .then(data => { return anotherAsyncFunction(data); }) .then(processedData => { console.log(processedData); }) .catch(error => { console.error(‘An error occurred:’, error); });
Async/Await: Syntactic Sugar for Promises
While Promises provide a significant improvement over callbacks for asynchronous JavaScript programming, they can still involve a bit of boilerplate code, especially when chaining multiple asynchronous operations. This is where async/await comes in. Introduced in ES7 (ECMAScript 2016) and later, async/await offers a cleaner, more synchronous-like syntax for working with Promises.
The Magic of async and await
The async keyword is used to declare an asynchronous function. This function can then use the await keyword before Promise-based operations. The await keyword pauses the execution of the async function until the preceding resolves. Once the Promise resolves, the await keyword returns the resolved value, and the async function continues execution.
Here’s an example of how async/await can be used to rewrite the previous promise chaining example:
JavaScript
async function fetchDataAndProcess() { try { const data = await fetchData(‘https://api.example.com/data’); const processedData = await anotherAsyncFunction(data); console.log(processedData); } catch (error) { console.error(error); } } fetchDataAndProcess();
Benefits of Async/Await:
• Improved Readability: Async/await makes asynchronous code appear more synchronous, resembling traditional sequential programming. This can significantly enhance code readability and maintainability.
• Error Handling: Similar to promises, async/await allows for error handling using a try…catch block. Any errors that are thrown within the async function or from awaited Promises will be caught by the catch block.
• Cleaner Chaining: Async/await eliminates the need for explicit chaining using .then(). The code reads more naturally, as if the asynchronous operations are happening sequentially.
Important Considerations:
• await Only Pauses the Async Function: It’s important to remember that await only pauses the execution of the current async function, not the entire JavaScript thread. Other parts of your application can continue to run while an await is in effect.
• Error Handling is Essential: Just like with Promises, proper error handling is crucial in asynchronous code. Always use try…catch blocks within async functions to handle potential errors from awaited Promises.
In Conclusion
Async/await builds upon Promises and provides a more concise and readable way to write asynchronous code in JavaScript. While Promises offer a robust foundation for asynchronous operations, async/await streamlines the process, making it feel more intuitive and synchronous-like. By mastering both Promises and async/await, you’ll be well-equipped to tackle asynchronous tasks in your JavaScript applications effectively.