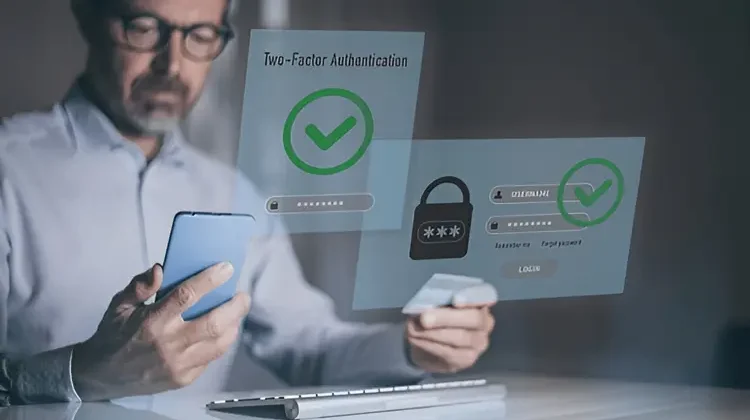
Authentication and Authorization in Back-End Development: The Gatekeepers of Your App’s Security
In the dynamic world of web applications, security is a constant concern. Back-end development, the hidden engine powering user interactions, serves as the central repository for sensitive data and functionalities. Ensuring only allowed users can access and manipulate this data is crucial. This two-step process, often referred to as Authentication and Authorization, forms the foundation for a secure back-end environment.
Understanding the Difference: Authentication vs. Authorization
While both terms are intertwined, Authentication and Authorization serve distinct purposes:
- Authentication: This process verifies a user’s claimed identity. Imagine a high-security building–the guard at the entrance checks your ID to confirm you’re allowed to enter. In back-end development, authentication mechanisms make sure users are who they say they are. Popular methods include:
- Username and Password: This traditional approach requires users to give a username and password that is checked against a secure database.
- Social Login: Users leverage existing social media accounts (e.g., Facebook, Google) to streamline the login process, minimizing the need for separate credentials.
- API Keys: For machine-to-machine interactions (e.g., mobile apps communicating with servers), unique API keys serve as secure identifiers for allowed applications.
Once a user’s identity is confirmed through authentication, the backdrop grants them a token — a digital key representing their legitimacy. This token acts as a temporary pass, allowing access to specific functionalities within the application.
- Authorization: After verifying identity, authorization determines what actions a user can do within the system. Think of a library with different user types: patrons can borrow books, librarians can add new books and manage accounts, and administrators have full access to all functionalities. Authorization enforces similar access control within an application. Here are the common approaches:
- Role-Based Access Control (RBAC): This scheme assigns user roles (e.g., admin, editor, reader) with predefined permissions. Users can only do actions aligned with their assigned roles.
- Attribute-Based Access Control (ABAC): This more granular approach considers various factors beyond user roles, such as location, time of day, or specific data attributes, to decide access permissions.
The Synergy of Authentication and Authorization
Authentication and Authorization work in tandem to create a robust security system for back-end development. Authentication verifies “who” the user is, while authorization dictates “what” they can do. This layered approach ensures that only legitimate users have access to specific functionalities and data, safeguarding sensitive information and preventing unauthorized actions.
Benefits of Implementing Authentication and Authorization
Effective Authentication and Authorization offer a multitude of benefits for back-end development:
- Enhanced Security: By verifying user identity and restricting access based on permissions, unauthorized breaches, and data manipulation are reduced.
- Improved User Experience: Streamlined login processes with secure mechanisms foster trust and confidence among users.
- Data Integrity: Authorized access controls make sure data is only accessible and modifiable by designated users, maintaining the accuracy and consistency of sensitive information.
- Scalability and Flexibility: Implementing modular authentication and authorization systems allows for easier scaling of user management and access control as applications evolve.
Building a Secure Back-End: Best Practices
When implementing Authentication and Authorization in your back-end development, consider these best practices to fortify your application’s security posture:
- Secure Storage and Transmission:
- Passwords: Never store passwords in plain text. Utilize strong hashing algorithms (e.g., bcrypt, Argon2) to encrypt passwords before storing them in your database. These algorithms create a one-way transformation, making it virtually impossible to decrypt the original password.
- Tokens: Implement secure transmission protocols like HTTPS (Hypertext Transfer Protocol Secure) for all communication involving user credentials and tokens. HTTPS encrypts data in transit, protecting it from eavesdropping or man-in-the-middle attacks.
- Robust Token Management:
- Expiration Times: Assign a limited lifespan to tokens, ensuring they expire after a reasonable period of inactivity. This mitigates the risk of unauthorized access even if a token is compromised.
- Refresh Tokens: Implement a two-token system. Utilize short-lived access tokens for resource access and longer-lived refresh tokens to obtain new access tokens without requiring constant re-authentication. However, ensure refresh tokens are stored securely and implement measures to prevent unauthorized refresh attempts.
- Regular Security Audits and Updates:
- Vulnerability Assessment: Regularly scan your application and server environments for potential security vulnerabilities. Leverage security testing tools and practices to identify and address weaknesses before they can be exploited.
- Software Updates: Stay updated on the latest security patches for your chosen libraries, frameworks, and server software.
- Input Validation and Sanitization:
- User Input: Malicious actors can inject harmful code into user input fields. Implement robust input validation to ensure users can only submit data in the expected format. Sanitize all user input before processing it, removing or escaping potentially harmful characters. This prevents techniques like SQL injection attacks, where attackers attempt to manipulate database queries through user input.
- Data Validation: Validate data coming from various sources, including external APIs or user uploads. Ensure data adheres to defined formats and expected values to prevent unexpected behavior or security vulnerabilities.
- Principle of The Least Privilege:
- Minimize Permissions: Grant users the minimum level of access necessary to perform their designated tasks. Avoid granting broad permissions unless required. This principle reduces the potential damage caused by compromised accounts or accidental misuse of functionalities.
- Secure Coding Practices:
- Coding Standards: Adhere to secure coding practices and guidelines specific to your chosen programming language and frameworks. These guidelines often address common vulnerabilities and provide recommendations for secure coding techniques.
- Security Libraries and Frameworks: Leverage established security libraries and frameworks that offer built-in functionalities for common tasks like user authentication, authorization, and data encryption. These tools can streamline development and reduce the risk of introducing security vulnerabilities through custom code.
- Secure Session Management:
- Session Management: Implement secure session management techniques to maintain user sessions. Utilize secure session IDs and avoid storing sensitive data within sessions as they can be vulnerable to hijacking. Consider session timeouts to automatically terminate inactive sessions after a predefined period.
- Logging and Monitoring:
- Comprehensive Logging: Maintain detailed logs of user activity, system events, and security-related incidents. Log data like login attempts, access requests, and any suspicious activity. These logs provide valuable insights for security analysis and incident detection.
- Security Monitoring: Implement security monitoring tools that can analyze logs in real time, identifying anomalies and potential security threats. These tools can alert you to suspicious activity, so you can take prompt action.
- User Education and Awareness:
- Strong Password Policies: Enforce strong password policies that require users to create complex passwords with a combination of uppercase and lowercase letters, numbers, and special characters. Encourage users to avoid password reuse across different applications.
- Security Best Practices: Educate users on security best practices like avoiding phishing attempts and being cautious about opening unknown links or attachments. User awareness can significantly reduce the risk of social engineering attacks that target human vulnerabilities.
- Defense in Depth:
- Layered Security: Don’t rely on a single security measure. Implement a layered approach, incorporating multiple security controls like authentication, authorization, input validation, and secure coding practices. This creates a strong defense against potential attacks, making it more difficult for attackers to breach your system.
Conclusion
By following these best practices and staying updated on evolving security threats, you can build a secure back-end that safeguards your application’s data and functionalities. Remember, security is an ongoing process, not a one-time fix. Regularly review your security posture, implement updates, and conduct security audits to maintain a robust defense against potential security breaches.